Android のBluetooth接続のコードを書いてみました。
BLE 接続の基礎。覚える用語は何かがよくわかる➡︎クラゲさんの記事
kotlin
Central = スマホ Android
端末をセントラルとします。
Peripheral = BLE機器
今回はイヤホンの接続方式です。
セントラルに自身の存在を知らせます。
セントラルと接続後にデータのやり取りを行います。
コピペすれば動くかもサンプル
MainActivity.kt
package com.example.bluetoothsample import BluetoothConnect import android.bluetooth.BluetoothAdapter import android.bluetooth.le.BluetoothLeScanner import android.bluetooth.le.ScanCallback import android.bluetooth.le.ScanResult import android.os.Bundle import android.widget.Toast import androidx.appcompat.app.AppCompatActivity import kotlinx.android.synthetic.main.activity_main.* var mBluetoothLeScanner: BluetoothLeScanner? = null var mScanCallback: ScanCallback? = null class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) button.setOnClickListener{ val bluetoothAdapter: BluetoothAdapter? = BluetoothAdapter.getDefaultAdapter() // Bluetoothサポートしているかのチェック if (bluetoothAdapter == null) { Toast.makeText(this, "Bluetooth未サポート", Toast.LENGTH_SHORT) finish() } mBluetoothLeScanner = bluetoothAdapter?.bluetoothLeScanner // kotlin プロパティ優先なのでメソッド使うな mScanCallback = initCallbacks() println(mScanCallback.toString()) println("mScanCallback") // スキャンの開始 mBluetoothLeScanner?.startScan(mScanCallback) // スキャンの停止 // mBluetoothLeScanner?.stopScan(mScanCallback) } } private fun initCallbacks(): ScanCallback? { return object : ScanCallback() { override fun onScanResult( callbackType: Int, result: ScanResult ) { super.onScanResult(callbackType, result) // デバイスが見つかった! if (result != null && result.device != null) { // リストに追加などなどの処理をおこなう //addDevice(result.getDevice(), result.getRssi()); println(result.getDevice()) println(result.getRssi()) } return } } } }
AndroidManifest.xml
<uses-permission android:name="android.permission.BLUETOOTH" /> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> <uses-feature android:name="android.hardware.bluetooth" />
詰まった所
Manifest に不足
AndroidManifest.xml
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
ScanFilterとScanSettings に nullを渡してはいけない
// スキャンの開始 // null渡すとクラッシュしました mBluetoothLeScanner?.startScan(null,null,mScanCallback)
⬇️
// スキャンの開始 mBluetoothLeScanner?.startScan(mScanCallback)
端末の設定→アプリ→権限 で位置情報を許可
参考
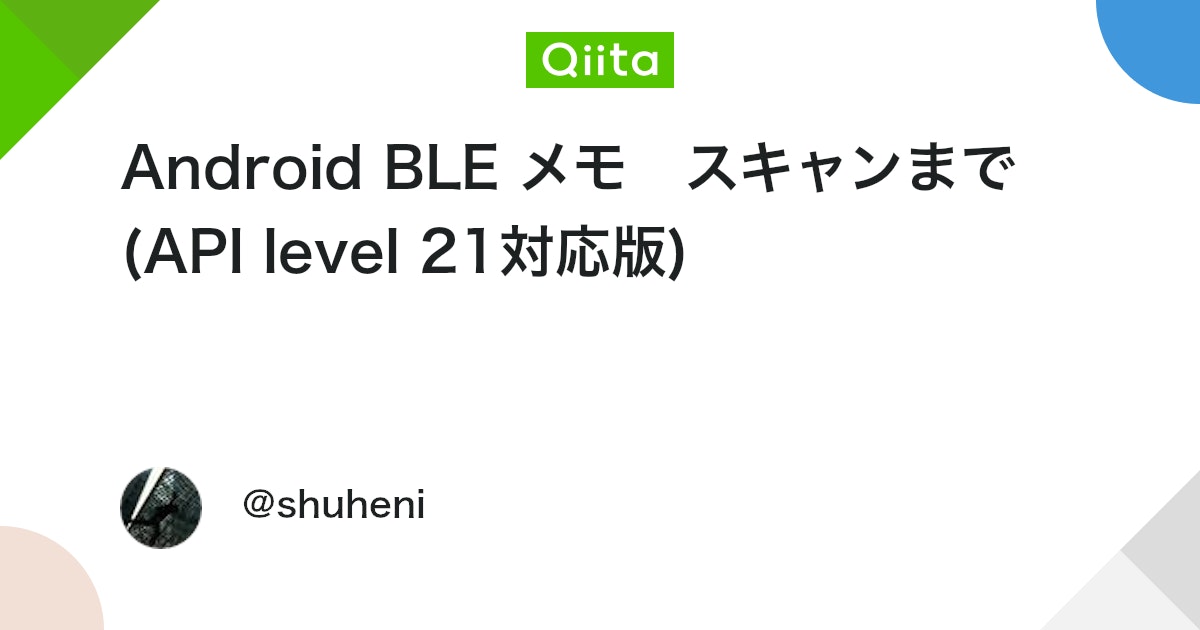
Android BLE メモ スキャンまで (API level 21対応版) - Qiita
AndroidでBLEを動作させるための備忘録として記載します。自分で理解できることを優先していますので、不足分は補完してくださいませ。。。マニュフェストへのパーミッション追加AndroidM…
GitHub - makotoishida/android_bluetooth_kotlin_demo: Android Bluetooth Demo in Kolin
Android Bluetooth Demo in Kolin. Contribute to makotoishida/android_bluetooth_kotlin_demo development by creating an account on GitHub.
エラーと解決方法
Unresolved reference: databinding
android { compileSdkVersion 29 //これと dataBinding { enabled = true } } dependencies { //これを追加 apply plugin: 'kotlin-kapt' }
コメント